반응형
1. 이제 뮤지컬 데이터를 DB에 INSERT까지 했으니 목록을 SELECT할 차례!!
2. 이번에 개인 프로젝트를 하는 목적은 여러 명이서만 해봤던 프로젝트 진행 과정을 처음부터 끝까지
3. 내 힘으로 하면서 공부하는 목적이 제일 크기 때문에 엄청 큰 규모로 할 생각도 없고
4. 일단 혼자 힘으로 해결하는 데에 의미가 가장 크다.
5. 그래서 프론트단은 타임리프(Thymeleaf) + 부트스트랩을 사용하기로 했다.
6. 🤍DTO, Service, Controller, Repository 작성
7. -먼저 Entity와 별개로 DTO를 추가로 작성해 주었다.
8. JPA로 처리할 때 변경이 적은 Entity 대신 DTO 객체를 만들어 원하는 컬럼의 기능만 뽑고 데이터를 교환하기 위해서.
9.
- MusicalDTO
1
2
3
4
5
6
7
8
9
10
11
12
13
|
@Data
@Builder
@NoArgsConstructor
@AllArgsConstructor
public class MusicalDTO {
private Long mcode;
private String mt20id; //뮤지컬코드
private String prfnm; //공연명
private String fcltynm; //공연장명
private String poster; //포스터 경로
private String prfstate; //공연 중 상태
}
|
cs |
- Entity를 DTO로, DTO를 Entity로 변환하는 코드
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
|
default MusicalDTO entitiesToDTO(Musical musical){
MusicalDTO musicalDTO = MusicalDTO.builder()
.mcode(musical.getMcode())
.prfnm(musical.getPrfnm())
.fcltynm(musical.getFcltynm())
.poster(musical.getPoster())
.prfstate(musical.getPrfstate())
.build();
return musicalDTO;
}
default Map<String,Object> dtoToEntity(MusicalDTO musicalDTO){
Map<String,Object> entityMap = new HashMap<>();
Musical musical = Musical.builder()
.mcode(musicalDTO.getMcode())
.prfnm(musicalDTO.getPrfnm())
.fcltynm(musicalDTO.getFcltynm())
.poster(musicalDTO.getPoster())
.prfstate(musicalDTO.getPrfstate())
.build();
entityMap.put("musical",musical);
return entityMap;
}
|
cs |
- MusicalServiceImpl
1
2
3
4
5
6
7
|
@Override
public PageResultDTO<MusicalDTO,Object[]> getList(PageRequestDTO requestDTO){
Pageable pageable = requestDTO.getPageable(Sort.by("mcode").descending());
Page<Object[]> result = musicalRepository.getListPage(pageable);
return new PageResultDTO<>(result);
}
|
cs |
- MusicalController
1
2
3
4
5
|
@GetMapping("list")
public void list(Model model, PageRequestDTO pageRequestDTO, Musical musical){
model.addAttribute("result",musicalService.getList(pageRequestDTO));
}
|
cs |
- MusicalRepository - SELECT 구문
1
2
3
4
5
6
7
8
9
10
11
12
13
|
package com.jungahzzzang.musicalcommunity.musical.repository;
import com.jungahzzzang.musicalcommunity.musical.domain.Musical;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.data.jpa.repository.Query;
public interface MusicalRepository extends JpaRepository<Musical,Long> {
@Query("SELECT m FROM Musical m")
Page<Object[]> getListPage(Pageable pageable);
}
|
cs |
10. 여기까지 작성하면 백쪽 코드는 끝이고. 이제 프론트를 구현할 차례!
11. 🤍Bootswatch 부트스트랩
12. 부트스트랩 템플릿 사이트는 엄청 많은데 나는 이번에 Bootswatch에서 제공하는 템플릿을 써보기로 했다.
13. https://bootswatch.com/
Bootswatch: Free themes for Bootstrap
Customizable Changes are contained in just two SASS files, enabling further customization and ensuring forward compatibility.
bootswatch.com
14. 이곳에서는 형식은 비슷하고 색상만 조금 다른 다양한 테마를 제공하는데,
15. 내 프로젝트가 게시판 기능을 주로 갖고 있는 뮤지컬 커뮤니티라
16. 어울릴 것 같다고 생각했다. 나는 이중 Minty 테마를 써보기로 했다.
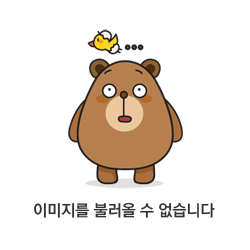
17.
18.
19. 부트스트랩을 사용하기 위해 별도로 다운받을 필요는 없고 아래 CDN 링크만 추가해 주면 된다.
<html />
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootswatch@4.5.2/dist/minty/bootstrap.min.css" integrity="sha384-H4X+4tKc7b8s4GoMrylmy2ssQYpDHoqzPa9aKXbDwPoPUA3Ra8PA5dGzijN+ePnH" crossorigin="anonymous">
20. 그리고 홈페이지에 설명이 매우 잘되어 있어서 그대로 소스를 긁어와서 원하는 위치에 추가해주면 된다.
21. 나는 Navbar와 Tables 을 가져오기로 했다.
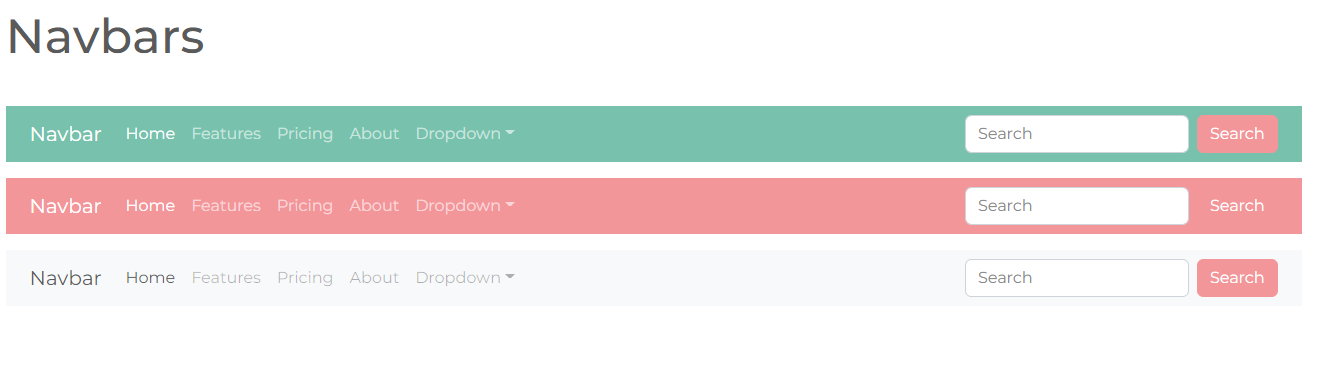
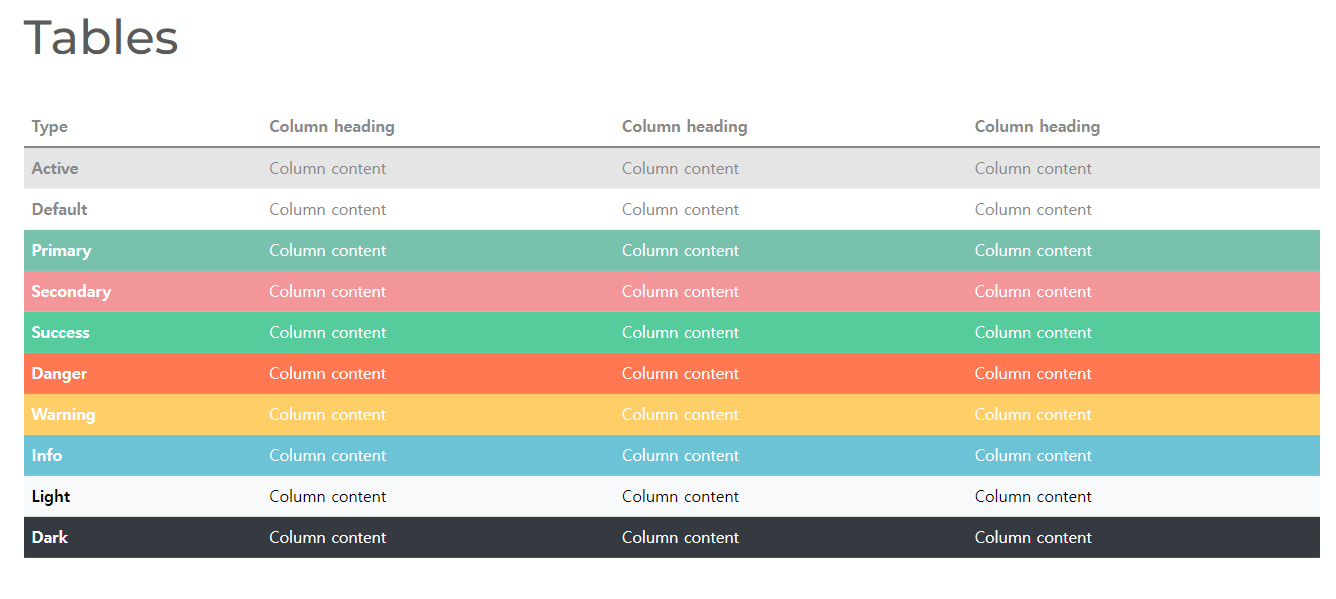
22. 마음에 드는 부분을 선택하면 아래와 같이 소스 코드를 제공하고 있어서 이걸 가져와서 활용하면 된다.
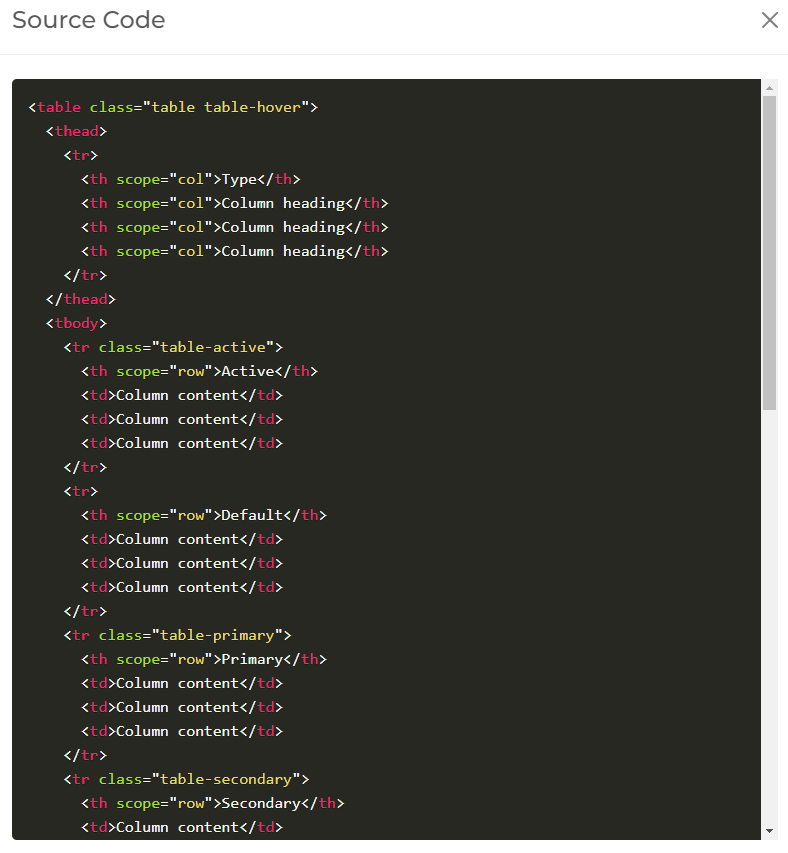
23. 간단하게 list.html을 타임리프 문법으로 작성해 주고 실행을 해보면
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
|
<table class="table table-hover">
<thead>
<tr class="table-primary">
<th scope="col">#</th>
<th scope="col">공연명</th>
<th scope="col">공연장</th>
<th scope="col">포스터</th>
<th scope="col">공연 상태</th>
</tr>
</thead>
<tbody>
<tr th:each="dto : ${result.dtoList}" class="table-light">
<th scope="row">
<!-- <a th:href="@{/musical/read(mcode = ${dto.mcode},page=${result.page})}">-->
[[${dto.mcode}]]
<!-- </a>-->
</th>
<td>[[${dto.prfnm}]]</td>
<td>[[${dto.fcltynm}]]</td>
<td><img th:src="${dto.poster}" style="width: 100px;"></td>
<td>[[${dto.prfstate}]]</td>
</tr>
</tbody>
</table>
<ul class="pagination h-100 justify-content-center align-items-center">
<li class="page-item " th:if="${result.prev}">
<a class="page-link" th:href="@{musical/list(page= ${result.start -1})}" tabindex="-1">Previous</a>
</li>
<li th:class=" 'page-item ' + ${result.page == page?'active':''} " th:each="page: ${result.pageList}">
<a class="page-link" th:href="@{musical/list(page = ${page})}">
[[${page}]]
</a>
</li>
<li class="page-item" th:if="${result.next}">
<a class="page-link" th:href="@{musical/list(page= ${result.end + 1} )}">Next</a>
</li>
</ul>
|
cs |
24. Select 구문이 잘 돌고
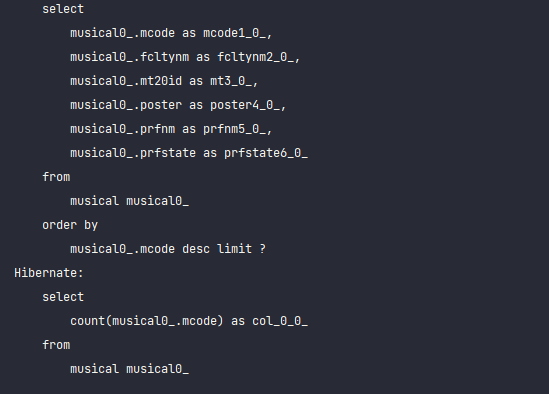
25. 화면도 잘 출력되었다.
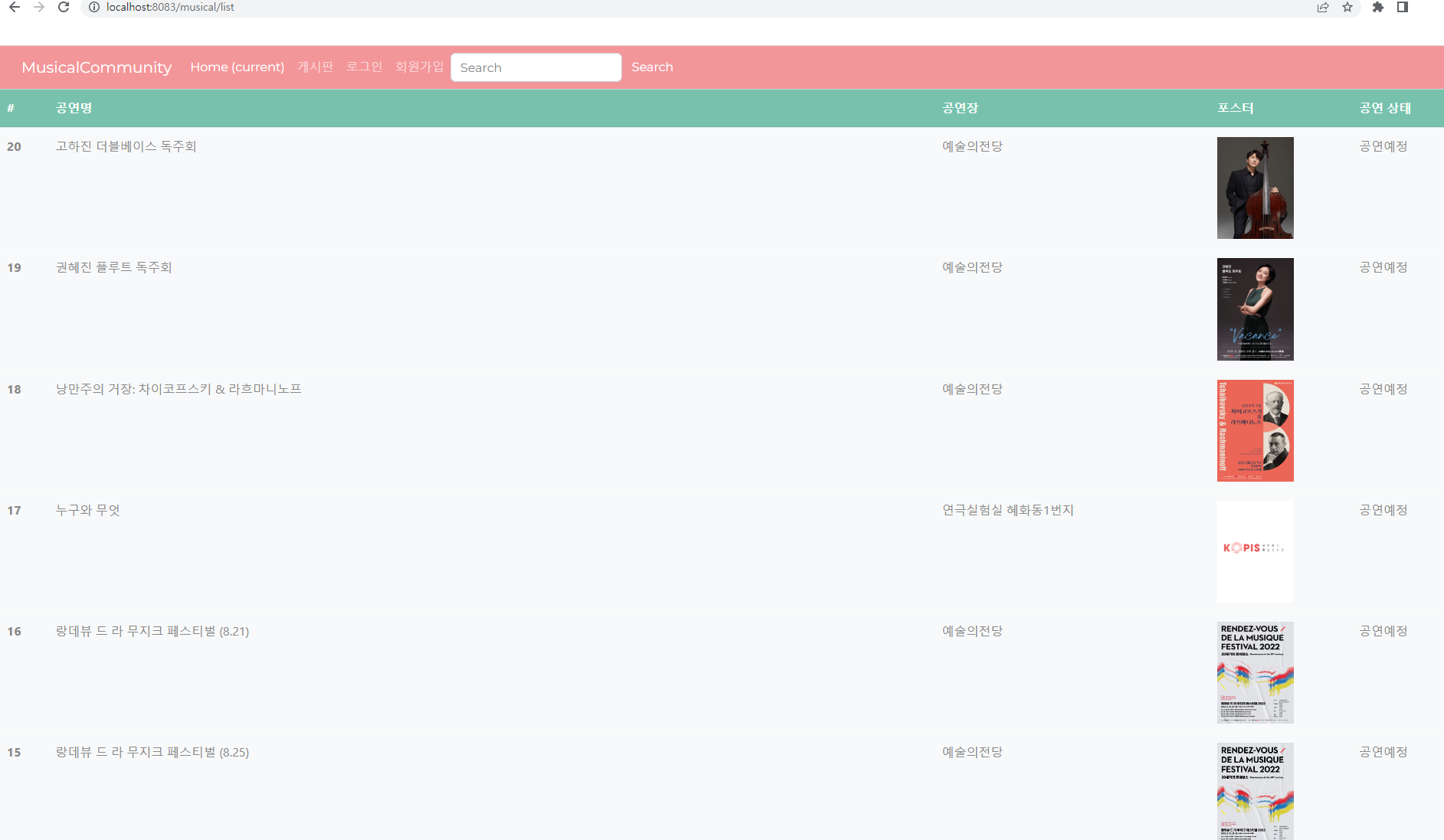
반응형
'💻 my code archive > 🌈Project' 카테고리의 다른 글
[RN 프로젝트] #1 개발 환경 구축, 프로젝트 디렉토리 구조 잡기 (0) | 2022.10.05 |
---|---|
[개인 프로젝트] 스프링 시큐리티(Spring Security), OAuth2 네이버 카카오 로그인 API 사용하기 (0) | 2022.06.28 |
[개인 프로젝트] KOPIS 공연 Open API 가져오기, XML JSON 파싱, DB 저장, 스프링 배치(Spring Batch) (4) | 2022.06.24 |
[개인 프로젝트] 뮤지컬 커뮤니티 만들기 - 준비 (0) | 2022.06.23 |
프로젝트 기록 - 마켓컬리 클론코딩, 레시피 게시판을 추가한 온라인 쇼핑몰 RecipeToYou 구축하기 (0) | 2022.03.10 |