POST 방식의 인코딩 방식에 따른 데이터 전송
-파일 업로드는 <form> 태그를 통해 파라미터를 전송하는 것과 같다.
-POST 방식은 전송하려는 데이터의 형식에 따라 두 가지 인코딩 방식 사용 가능 (아래에서 설명)
--uploadForm.jsp
--<input> 태그의 type 속성을 "file"로 지정
--"multipart/form-data" 인코딩 방식을 통해 파일 전송
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
|
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%@ taglib prefix="fmt" uri="http://java.sun.com/jsp/jstl/fmt" %>
<%
request.setCharacterEncoding("utf-8");
%>
<c:set var="contextPath" value="${pageContext.request.servletContext.contextPath }"></c:set>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>파일 업로드</title>
</head>
<body>
<%-- enctype의 속성값 multipart/form-data : 모든 문자를 인코딩하지 않음.
<form>태그를 통해 파일을 서버로 전송할 때 주로 사용 --%>
<form action="${contextPath }/upload.do" method="post" enctype="multipart/form-data">
파일1: <input type="file" name="file1"><br>
파일2: <input type="file" name="file2"><br>
매개변수1: <input type="text" name="param1"><br>
매개변수2: <input type="text" name="param2"><br>
매개변수3: <input type="text" name="param2"><br>
<input type="submit" value="업로드">
</form>
</body>
</html>
|
cs |
--FileUpload.java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
|
import java.io.File;
import java.io.IOException;
import java.util.List;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.commons.fileupload.FileItem;
import org.apache.commons.fileupload.disk.DiskFileItemFactory;
import org.apache.commons.fileupload.servlet.ServletFileUpload;
/**
* Servlet implementation class FileUpload
*/
@WebServlet("/upload.do")
public class FileUpload extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doHandle(request, response);
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doHandle(request, response);
}
protected void doHandle(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("utf-8");
String encoding = "utf-8";
File currenDirPath = new File("C:\\workspace-jsp\\FileRepo"); //업로드할 파일 경로 지정
DiskFileItemFactory factory = new DiskFileItemFactory();
factory.setRepository(currenDirPath); //파일 경로 설정
factory.setSizeThreshold(1024*1024); //최대 업로드 파일 크기 설정
ServletFileUpload upload = new ServletFileUpload(factory);
try {
List<FileItem> items = upload.parseRequest(request); //request 객체에서 매개변수를 List로 가져옴.
for(int i=0;i<items.size();i++) {
FileItem fileItem = items.get(i); //파일 업로드 창에서 업로드된 항목을 하나씩 가져옴.
if(fileItem.isFormField()) {
//폼 필드이면 전송된 매개변수 값을 출력함
System.out.println(fileItem.getFieldName() +"="+fileItem.getString(encoding));
}else {
System.out.println("파라미터명:"+fileItem.getFieldName());
System.out.println("파일명:"+fileItem.getName());
System.out.println("파일크기:"+fileItem.getSize()+"bytes");
if(fileItem.getSize() > 0) {
int idx = fileItem.getName().lastIndexOf("\\");
if(idx== -1) {
idx = fileItem.getName().lastIndexOf("/");
}
String fileName = fileItem.getName().substring(idx+1);
File uploadFile = new File(currenDirPath+"\\"+fileName);
//업로드한 파일 이름으로 저장소에 파일을 업로드함.
fileItem.write(uploadFile);
}
}
}
}catch (Exception e) {
System.out.println("파일 업로드 중 오류 발생");
e.printStackTrace();
}
}
}
|
cs |
--download.jsp
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
|
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
request.setCharacterEncoding("utf-8");
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>파일 다운로드 요청</title>
</head>
<body>
<form action="result.jsp" method="post">
<%-- 다운로드할 파일 이름을 매개변수로 전달함 --%>
<input type="hidden" name="param1" value="AirPods(3).png">
<input type="hidden" name="param2" value="airpods.png">
<input type="submit" value="이미지 다운로드">
</form>
</body>
</html>
|
cs |
--FileDownload.java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
|
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.OutputStream;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/**
* Servlet implementation class FileDownload
*/
@WebServlet("/download.do")
public class FileDownload extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
dohandle(request, response);
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
dohandle(request, response);
}
private void dohandle(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("utf-8");
response.setContentType("text/html; charset=utf-8");
String fileRepo = "C:\\workspace-jsp\\FileRepo";
String fileName = request.getParameter("fileName");
System.out.println("fileName= " + fileName);
OutputStream out = response.getOutputStream();
String downFile = fileRepo +"\\"+ fileName;
File f = new File(downFile);
//파일 다운로드 하기 위해 설정
response.setHeader("Cache-Control", "no-cache");
response.addHeader("Content-disposition", "attachment; fileName=" +fileName );
FileInputStream in = new FileInputStream(f);
byte[] buffer = new byte[1024 * 8];
while(true) {
int count = in.read(buffer);
if (count == -1) break;
out.write(buffer, 0, count);
}
in.close();
out.close();
}
}
|
cs |
COS 라이브러리 이용한 파일 업로드, 다운로드 구현
■ COS 라이브러리 다운로드 링크 : http://www.servlets.com/cos/
Servlets.com | com.oreilly.servlet
www.servlets.com
■ 다운로드 파일
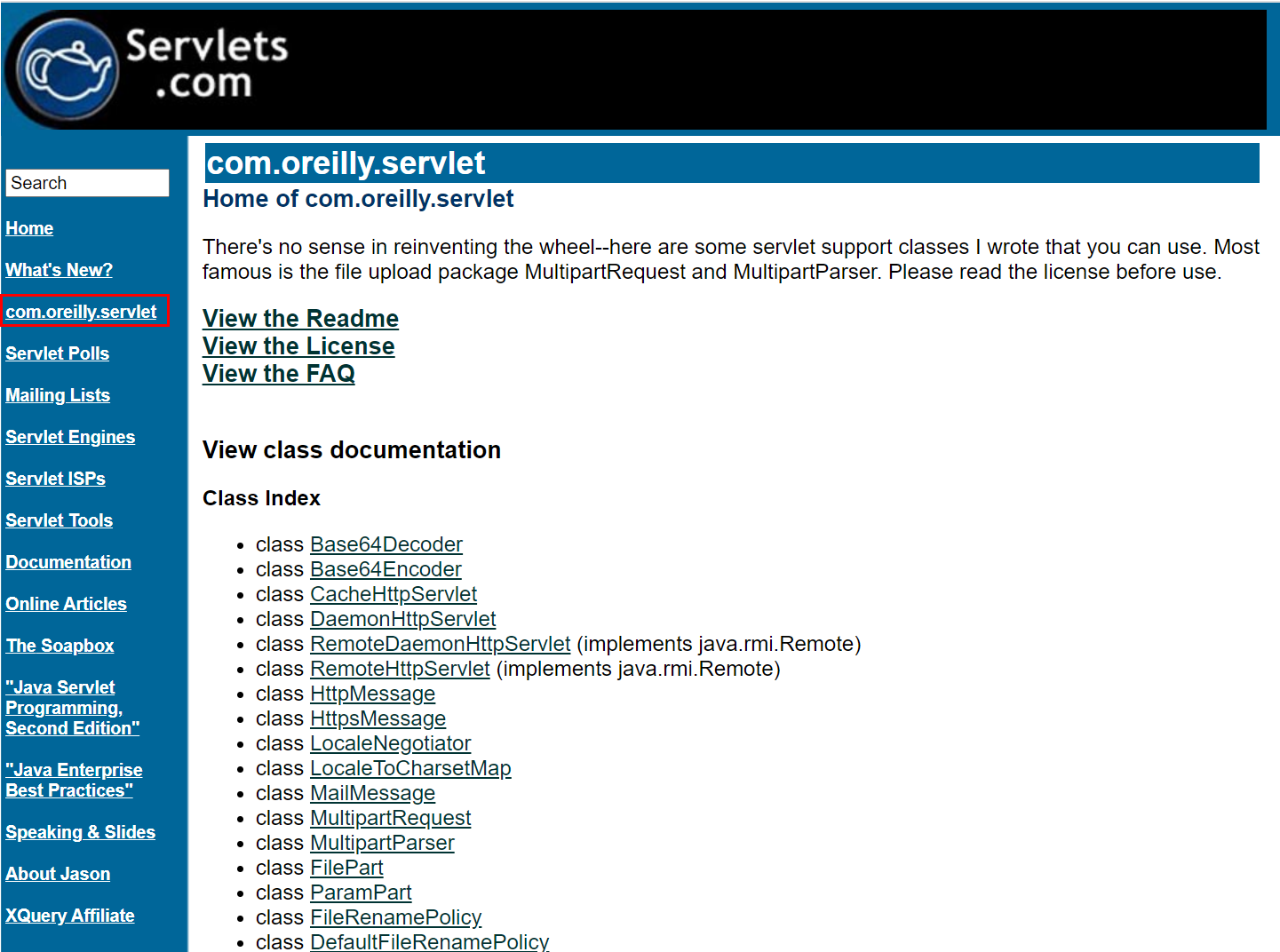
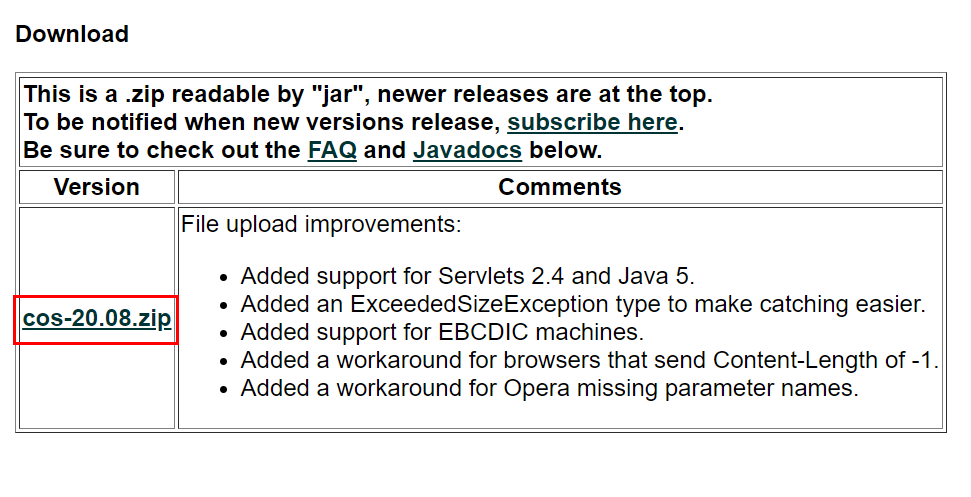
JSP 파일 업로드
1. 파일 업로드 관련 API
-DiskFileItemFactory
-setRepository() : 파일을 저장할 디렉토리 설정
-setSizeThreadhold() : 최대 업로드 가능 파일 크기 설정
-ServletFileUpload
-parseRequest() : 전송된 매개변수를 List 객체로 얻음
-getItemIterator() : 전송된 매개변수를 Iterator 타입으로 얻음.
2. 순서
1)라이브러리 추가
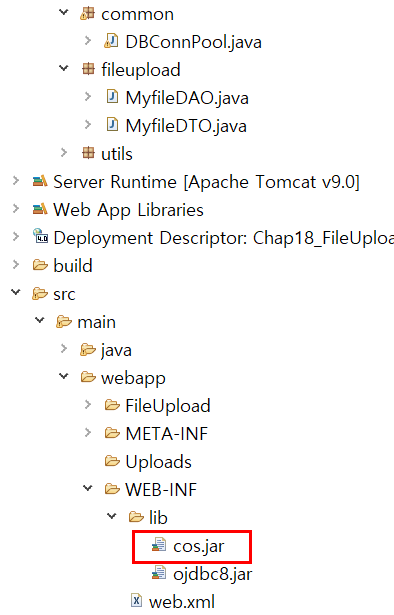
2)파일 업로드
-화면 폼 작성
■ application/x-www-form-urlencoded (기본값)
:모든 문자를 서버로 전송하기 전에 인코딩함.
■ multipart/form-data
:모든 문자를 인코딩하지 않음, <form>태그를 통해 파일을 서버로 전송할 때 주로 사용함.
■ text/plain
:공백 문자(space)만 "+" 기호로 변환하고 나머지 문자는 인코딩하지 않음.
--fileUploadMain.jsp
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
|
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Insert title here</title>
<script>
function validationForm(form){
if(fileForm.name.value==""){
alert("작성자를 입력하세요.");
form.name.focus();
return false;
}
if(fileForm.title.value==""){
alert("제목를 입력하세요.");
form.name.focus();
return false;
}
if(fileForm.attachedFile.value==""){
alert("첨부파일은 필수입니다.");
form.name.focus();
return false;
}
}
</script>
</head>
<body>
<h3>파일 업로드</h3>
<span style="color:red;">${errorMessage }</span>
<form action="UploadProcess.jsp" method="post" name="fileForm"
enctype="multipart/form-data" onsubmit="return validationForm(this)">
작성자 : <input type="text" name="name" value="ezen"/><br/>
제목: <input type="text" name="title"><br/>
카테고리(선택사항):<input type="checkbox" name="category" value="사진" checked>사진
<input type="checkbox" name="category" value="과제" >과제
<input type="checkbox" name="category" value="사진" >슬라이드
<input type="checkbox" name="category" value="사진" >음원<br/>
첨부파일:<input type="file" name="attachedFile"/><br/>
<input type="submit" value="전송하기"/>
</form>
</body>
</html>
|
cs |
--UploadProcess.jsp
파일 업로드 처리
1)MultipartRequest 객체 생성
MultipartRequest mr = new MultipartReques
(HttpServletRequest request, //내장객체
String saveDirectory, //파일이 저장될 물리적인 경로
int maxPostSize, //업로드 파일의 최대 용량
String encoding) //인코딩 방식
2)새로운 파일명 생성("업로드 일시.확장자")
3)파일명 변경
4)다른 폼값 처리
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
|
<%@page import="kr.co.ezenac.fileupload.MyfileDAO"%>
<%@page import="kr.co.ezenac.fileupload.MyfileDTO"%>
<%@page import="java.io.File"%>
<%@page import="java.util.Date"%>
<%@page import="java.text.SimpleDateFormat"%>
<%@page import="com.oreilly.servlet.MultipartRequest"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
String saveDirectory = application.getRealPath("/Uploads"); //저장할 디렉토리
int maxPostSize = 1024 * 1000; //파일 최대 크기(1MB)로 설정
String encoding = "UTF-8"; //인코딩 방식
try{
// 1)MultipartRequest 객체 생성
MultipartRequest mr = new MultipartRequest(request, saveDirectory, maxPostSize, encoding);
// 2)새로운 파일명 생성("업로드 일시.확장자")
String filename = mr.getFilesystemName("attachedFile"); //현재 파일 이름
String ext = filename.substring(filename.lastIndexOf(".")); //파일 확장자
String now = new SimpleDateFormat("yyyyMMdd_HmsS").format(new Date());
String newFileName = now + ext; //새로운 파일 이름("업로드일시.확장자")
// 3)파일명 변경
//File.separator : 특수 기호, 서버 OS에 경로 표시 방법 다르기 때문에
// =>환경에 상관없이 동작하게함.
File oldFile = new File(saveDirectory + File.separator + filename);
File newFile = new File(saveDirectory + File.separator + newFileName);
oldFile.renameTo(newFile);
// 4)다른 폼값 처리
String name = mr.getParameter("name");
String title = mr.getParameter("title");
String[] category = mr.getParameterValues("category");
StringBuffer cateBuf = new StringBuffer();
if(category == null){
cateBuf.append("선택 없음");
}else{
for(String s : category){
cateBuf.append(s+", ");
}
}
// 5)DTO 생성
MyfileDTO dto = new MyfileDTO();
dto.setName(name);
dto.setTitle(title);
dto.setCategory(cateBuf.toString());
dto.setOfile(filename);
dto.setSfile(newFileName);
// 6)DAO의 insertFile(dto)
MyfileDAO dao = new MyfileDAO();
dao.insertFile(dto);
dao.close();
// 7)파일 목록 jsp 화면으로 리다이렉트
response.sendRedirect("fileList.jsp");
}catch(Exception e){
System.out.println("파일 업로드 에러");
e.printStackTrace();
request.setAttribute("errorMessage", "파일 업로드 오류");
request.getRequestDispatcher("fileUploadMain.jsp").forward(request, response);
}
%>
|
cs |
--fileList.jsp
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
|
<%@page import="java.net.URLEncoder"%>
<%@page import="kr.co.ezenac.fileupload.MyfileDTO"%>
<%@page import="java.util.List"%>
<%@page import="kr.co.ezenac.fileupload.MyfileDAO"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>DB에 등록된 파일 목록보기</title>
</head>
<body>
<h2>DB에 등록된 파일 목록 보기</h2>
<a href="fileUploadMain.jsp">파일 등록하기</a>
<%
MyfileDAO dao = new MyfileDAO();
List<MyfileDTO> fileLists = dao.myFileList();
dao.close();
%>
<table border="1">
<tr>
<th>No</th><th>작성자</th><th>제목</th><th>카테고리</th>
<th>원본 파일명</th><th>저장된 파일명</th><th>작성일</th><th></th>
</tr>
<%
for(MyfileDTO f : fileLists){
%>
<tr>
<td><%=f.getIdx() %></td>
<td><%=f.getName() %></td>
<td><%=f.getTitle() %></td>
<td><%=f.getCategory() %></td>
<td><%=f.getOfile() %></td>
<td><%=f.getSfile() %></td>
<td><%=f.getPostdate() %></td>
<td><a href="download.jsp?oName=<%=URLEncoder.encode(f.getOfile(), "UTF-8") %>&sName=<%=URLEncoder.encode(f.getSfile(), "UTF-8") %>">[다운로드]</a>
</tr>
<%
}
%>
</table>
</body>
</html>
|
cs |
--JSFunction.java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
|
import javax.servlet.jsp.JspWriter;
// 메시지 알림창을 띄운 후 명시한 URL로 이동함
/*
* msg : 알림창에 띄울 메시지
* url : 알림창을 닫은 후 이동할 페이지의 URL
* out : 자바스크립트 코드를 삽입할 출력
*/
public class JSFunction {
public static void alertLocation(String msg, String url, JspWriter out) {
try {
String script = ""
+ "<script>"
+ " alert('"+msg+"');"
+ " location.href='"+url+"'; "
+ "</script>";
out.println(script);
}
catch (Exception e) {}
}
public static void alertBack(String msg, JspWriter out) {
try {
String script = ""
+ "<script>"
+ " alert('"+msg+"');"
+ " history.back();"
+ "</script>";
out.println(script);
}
catch (Exception e) {}
}
}
|
cs |
-데이터베이스 테이블, 시퀀스 생성
컬럼명 | 데이터 타입 | null 허용 | 키 | 설명 |
idx | number | N | PK | 일렬번호, 기본키 |
name | varchar2(50) | N | 작성자 | |
title | varchar2(200) | N | 제목 | |
category | varchar2(30) | 카테고리 | ||
ofile | varchar2(100) | N | 원본 파일 이름 | |
sfile | varchar2(30) | N | 저장된 파일 이름 | |
postdate | date | N | 등록된 날짜 |
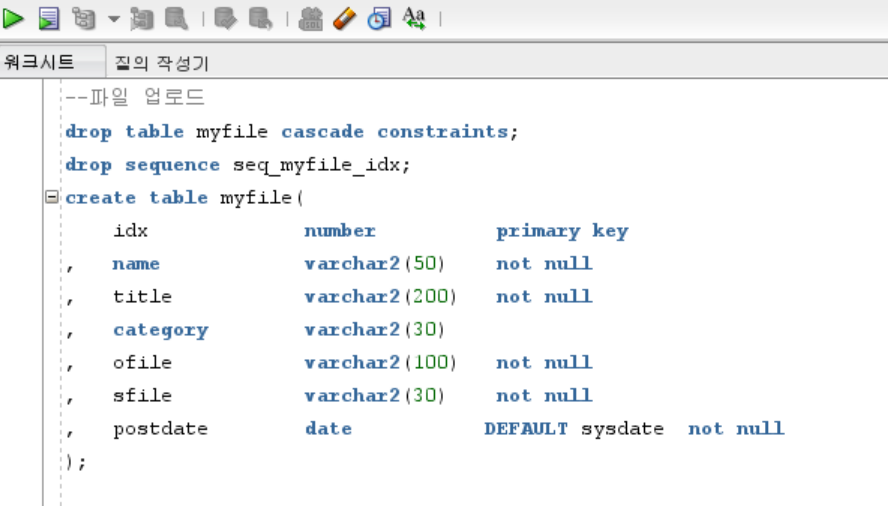
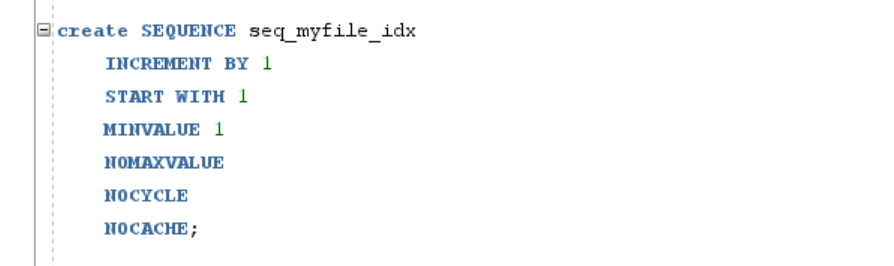
-DTO, DAO 작성
--MyfileDTO.java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
|
public class MyfileDTO {
private String idx;
private String name;
private String title;
private String category;
private String ofile;
private String sfile;
private String postdate;
public MyfileDTO() {
// TODO Auto-generated constructor stub
}
public String getIdx() {
return idx;
}
public void setIdx(String idx) {
this.idx = idx;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getCategory() {
return category;
}
public void setCategory(String category) {
this.category = category;
}
public String getOfile() {
return ofile;
}
public void setOfile(String ofile) {
this.ofile = ofile;
}
public String getSfile() {
return sfile;
}
public void setSfile(String sfile) {
this.sfile = sfile;
}
public String getPostdate() {
return postdate;
}
public void setPostdate(String postdate) {
this.postdate = postdate;
}
}
|
cs |
--DB 연동
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
|
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.Statement;
import javax.naming.Context;
import javax.naming.InitialContext;
import javax.naming.NamingException;
import javax.sql.DataSource;
public class DBConnPool {
public Connection con;
public Statement stmt;
public PreparedStatement pstmt;
public ResultSet rs;
//기본 생성자
public DBConnPool() {
//커넥션 풀(DataSource)얻기
try {
Context context = new InitialContext();
Context ctx = (Context)context.lookup("java:comp/env");
DataSource source = (DataSource)ctx.lookup("jdbc/oracle");
//커넥션 풀을 통해서 연결
con = source.getConnection();
System.out.println("DB 커넥션풀 연결 성공");
} catch (Exception e) {
System.out.println("DB 커넥션풀 연결 실패");
e.printStackTrace();
}
}
//연결 해제(자원 반납)
public void close() {
try {
if(rs!=null)
rs.close();
if(pstmt!=null)
pstmt.close();
if(con!=null)
con.close();
if(stmt!=null)
stmt.close();
System.out.println("DB 커넥션풀 자원 반납");
}catch (Exception e) {
System.out.println("DB 커넥션풀 자원 반납 실패");
e.printStackTrace();
}
}
}
|
cs |
--MyfileDAO.java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
|
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import kr.co.ezenac.common.DBConnPool;
public class MyfileDAO extends DBConnPool {
//새로운 게시물을 입력
public int insertFile(MyfileDTO dto) {
int applyResult = 0;
try {
String query = "INSERT INTO myfile (idx, name, title, category, ofile, sfile)"
+ "VALUES (seq_myfile_idx.nextval, ?, ?, ?, ?, ?)";
pstmt = con.prepareStatement(query);
pstmt.setString(1, dto.getName());
pstmt.setString(2, dto.getTitle());
pstmt.setString(3, dto.getCategory());
pstmt.setString(4, dto.getOfile());
pstmt.setString(5, dto.getSfile());
applyResult = pstmt.executeUpdate();
}catch (Exception e) {
System.out.println("insert 중 예외 발생");
}
return applyResult;
}
//파일 목록 반환
public List<MyfileDTO> myFileList(){
List<MyfileDTO> fileList = new ArrayList<>();
try {
String query = "SELECT * FROM myfile ORDER BY idx DESC";
pstmt=con.prepareStatement(query); //쿼리 준비
rs = pstmt.executeQuery(); //쿼리 실행
while(rs.next()) { //목록 안의 게시물 수만큼 반복
MyfileDTO dto = new MyfileDTO();
dto.setIdx(rs.getString(1));
dto.setName(rs.getString(2));
dto.setTitle(rs.getString(3));
dto.setCategory(rs.getString(4));
dto.setOfile(rs.getString(5));
dto.setSfile(rs.getString(6));
dto.setPostdate(rs.getString(7));
fileList.add(dto); //목록에 추가
}
} catch (SQLException e) {
System.out.println("SELECT 중 예외 발생");
e.printStackTrace();
}
return fileList;
}
}
|
cs |
-연동 완성
3)파일 다운로드
--download.jsp
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
|
<%@page import="java.io.OutputStream"%>
<%@page import="java.io.FileInputStream"%>
<%@page import="java.io.InputStream"%>
<%@page import="java.io.File"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%
String saveDirectory = application.getRealPath("/Uploads"); //저장할 디렉토리
String originalFileName = request.getParameter("oName");
String saveFileName = request.getParameter("sName");
try{
//파일 입력스트림 생성
File file = new File(saveDirectory, saveFileName);
InputStream inStream = new FileInputStream(file);
//한글 파일명 깨짐 방지
String client = request.getHeader("User-Agent");
if(client.indexOf("WOW64")==-1){
//getBytes("UTF-8")로 원본 파일명을 바이트 배열로 변환, ISO-8859-1 캐릭터 셋 문자열로 재생성함
originalFileName = new String(originalFileName.getBytes("UTF-8"), "ISO-8859-1");
}else{
//ie 경우 getBytes("KSC5601")을 이용하여 바이트 배열로 변환, 문자열로 재생성함
originalFileName = new String(originalFileName.getBytes("KSC5601"), "ISO-8859-1");
}
//파일 다운로드용 응답 헤더 초기화
response.reset();
//파일 다운로드 창을 띄우기 위한 콘텐츠 타입 지정
//octet-stream : 8비트 단위의 바이너리 데이터
response.setContentType("application/octet-stream");
//웹 브라우저에서 파일 다운로드창이 뜰 때 원본 파일명이 기본으로 입력되어 있도록 설정
response.setHeader("Content-Disposition", "attachment; filename=\""+originalFileName+"\"");
response.setHeader("Content-Length", ""+file.length() );
//출력 스트림 초기화--다른 JSP를 열면 출력 스트림 중복 생성 방지하기 위해 초기화함
out.clear();
//내장객체인 response로부터 새로운 출력스트림 생성
OutputStream outStream = response.getOutputStream();
byte[] b = new byte[(int)file.length()];
int readBuffer = 0;
while((readBuffer = inStream.read(b))>0){
outStream.write(b, 0, readBuffer);
}
//스트림 반납
inStream.close();
outStream.close();
}catch(Exception e){
//System.out.println("다운로드 중 예외 발생");
e.printStackTrace();
}
%>
|
cs |
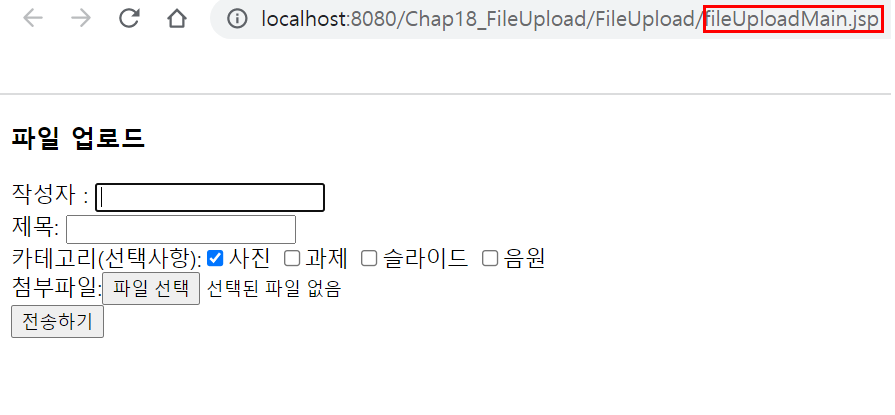
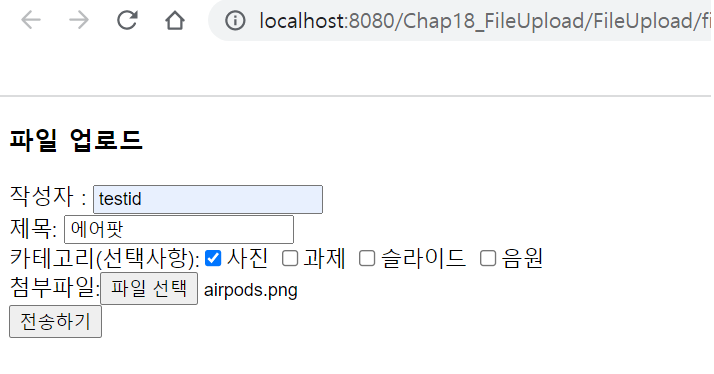
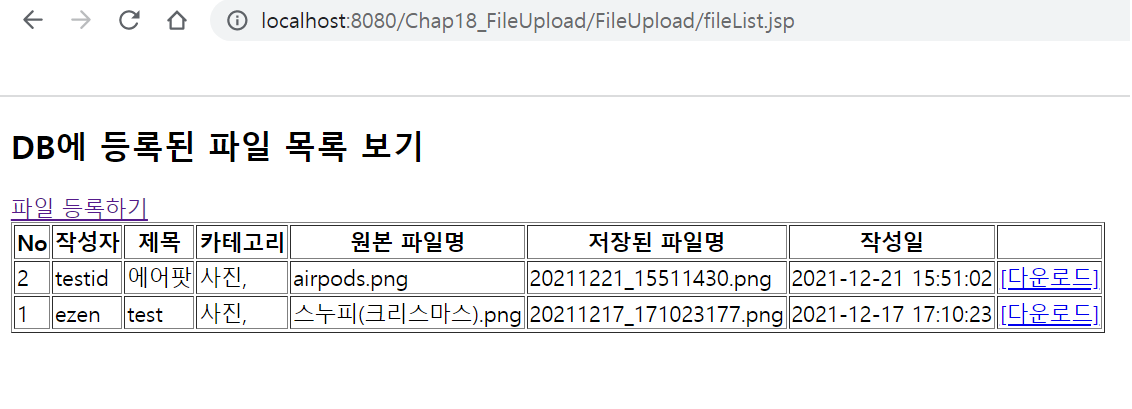
'📒 education archive > 📍Servlet, JSP' 카테고리의 다른 글
[국비학원 기록/JSP] JSTL - 국제화(다국어) 태그 라이브러리 (0) | 2021.12.16 |
---|---|
[국비학원 기록/JSP] JSTL - <c:url>, <c:redirect>,<c:out> 형식 (0) | 2021.12.16 |
[국비학원 기록/JSP] 커스텀 태그 JSTL 조건문, 반복문 - <c:set>, <c:if>, <c:forEach> (0) | 2021.12.15 |
[국비학원 기록/JSP] 표현 언어 EL, 연산자, 내장 객체, 자바 빈(Bean), ArrayList, HashMap 예제 (0) | 2021.12.14 |
[국비학원 기록/JSP] 액션태그(2)-useBean, setProperty, getProperty, 회원 가입 구현 (0) | 2021.12.10 |